you'll love perl then.Franky wrote:Well, I'm not saying that indenting isn't a good thing. I just don't like being forced to do it.
ZH's software thread
Moderator: General Mods
Re: I now have a better chance at becoming l33t
Code: Select all
int lowest = 0;
int highest = 0;
while ( true ) {
cout << "Enter number (0 to finish): ";
cin >> num;
if ( num == 0 ) {
return 0;
}
if ( num < lowest ) {
lowest = num;
} else if ( num > highest ) {
highest = num;
}
cout<<"Lowest number "<< lowest <<" and highest number "<< highest << endl;
}
And well done on your first C++ program[me].
-
- Seen it all
- Posts: 2302
- Joined: Mon Jan 03, 2005 5:04 pm
- Location: Germany
- Contact:
There are a lot of unwritten rules. Goto is bad, separate control code from implementation code, write small functions that do one thing, indendation is good, consistent indendation is better, etc.Franky wrote:Well, I'm not saying that indenting isn't a good thing. I just don't like being forced to do it.
Unless you know several languages, and know when to temporarily ignore the rules, you have no authority to bitch about them.
vSNES | Delphi 10 BPLs
bsnes launcher with recent files list
bsnes launcher with recent files list
Oh yeah, definitely.Goto is bad
Code: Select all
bool epicfail() {
bool success = false;
resource *a = open_file_source();
if(!a) goto This;
resource *b = open_file_dest();
if(!b) goto Code;
resource *c = allocate_memory();
if(!c) goto Is;
resource *d = get_exclusive_lock();
if(!d) goto Bad;
...
success = true;
release_exclusive_lock();
Bad:
free_memory();
Is:
close_file_dest();
Code:
close_file_source();
This:
return success;
}
This is so much better:
Code: Select all
bool make_java_proud() {
resource *a = open_file_source();
if(a)
{
resource *b = open_file_dest();
if(b)
{
resource *c = allocate_memory();
if(c)
{
resource *d = get_exclusive_lock();
if(d)
{
...
release_exclusive_lock();
free_memory();
close_file_dest();
close_file_source();
return true;
}
else
{
free_memory();
close_file_dest();
close_file_source();
return false;
}
}
else
{
close_file_dest();
close_file_source();
return false;
}
}
else
{
close_file_source();
return false;
}
}
else
{
return false;
}
}
-
- Seen it all
- Posts: 2302
- Joined: Mon Jan 03, 2005 5:04 pm
- Location: Germany
- Contact:
Ah, excellent case for exceptions!
Exeptions just look complicated (well, they are), unless you do something like this:
... which is not much easier to understand.
Code: Select all
function MakeJavaProud : Boolean;
begin
Result := False;
if (not OpenFileSource(a)) then Exit;
try
if (not OpenFileDest(b)) then Exit;
try
if (not AllocateMemory(c)) then Exit;
try
if (not GetExclusiveLock(d)) then Exit;
try
//...
Result := True;
finally
ReleaseExclusiveLock(d);
end;
finally
FreeMemory(c);
end;
finally
CloseFileDest(b);
end;
finally
CloseFileSource(a);
end;
end;
Code: Select all
function MakeJavaProud : Boolean;
begin
Result := False;
if (not OpenFileSource (a)) then Exit; try
if (not OpenFileDest (b)) then Exit; try
if (not AllocateMemory (c)) then Exit; try
if (not GetExclusiveLock(d)) then Exit; try
//...
Result := True;
finally ReleaseExclusiveLock(d); end;
finally FreeMemory (c); end;
finally CloseFileDest (b); end;
finally CloseFileSource (a); end;
end;
vSNES | Delphi 10 BPLs
bsnes launcher with recent files list
bsnes launcher with recent files list
Well, I've found something that (for now) makes Python better than C++.
Compare the two images of the same program, but written in different languages (python and C++). Click the thumbnails to see them fullsize:
C++ version:
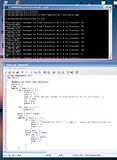
Python version:
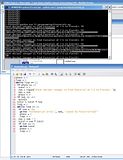
they are both of the program I posted that find the factorial of a number. One is in C++ (the one I originally posted), and the other is a version of it in python. As you can see, python displays large numbers, where are C++ (or more specifically, double variables) resort to standard form. Standard form is cool, but sometimes it displays a "rounded up" (i,e, completely innacurate) number (standard form can't do everything, you know).
So, for large numbers, python is currently best. Still, right now I'm favouring C++ much more than I favour python.
Compare the two images of the same program, but written in different languages (python and C++). Click the thumbnails to see them fullsize:
C++ version:
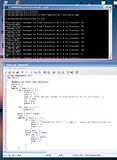
Python version:
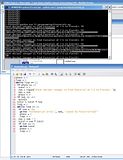
they are both of the program I posted that find the factorial of a number. One is in C++ (the one I originally posted), and the other is a version of it in python. As you can see, python displays large numbers, where are C++ (or more specifically, double variables) resort to standard form. Standard form is cool, but sometimes it displays a "rounded up" (i,e, completely innacurate) number (standard form can't do everything, you know).
So, for large numbers, python is currently best. Still, right now I'm favouring C++ much more than I favour python.
-
- ZSNES Shake Shake Prinny
- Posts: 5632
- Joined: Wed Jul 28, 2004 4:15 pm
- Location: PAL50, dood !
Yeah, you jackass. fclose and free and others don't die horribly if you pass them a null pointer, duh. And for those who do, wrap them in something that doesn't.byuu wrote:Oh yeah, definitely.Goto is bad
<epicfail()>
What kind of jackass writes code like that?
This is so much better:
<make_java_proud()>
:D
Forgot the obligatory ":D", for those who need it.
Code: Select all
bool use_brain()
{
bool ret = -1;
FILE *sauce_fp = fopen(input.fname, "rb");
FILE *dest_fp = fopen(output.fname, "rb");
char *buffer = (char *)malloc(BUFSIZE);
// ...
if (sauce_fp && dest_fp && buffer /* && ... */)
{
// stuff
ret = 0;
}
// ...
free(buffer);
fclose(dest_fp);
fclose(sauce_fp);
return (ret);
}
Unless you like goto, of course. This coming from a consummate ASM whore.
皆黙って俺について来い!!
Pantheon: Gideon Zhi | CaitSith2 | Nach | kode54
Code: Select all
<jmr> bsnes has the most accurate wiki page but it takes forever to load (or something)
Ok, so anyway I did that bin2dec and dec2bin program, in visual basic:
Decimal to Binary:
Binary to decimal:
So what do you guys think?
I'm going to be writing a few more programs in vb soon:
- I'll improve upon the dec2bin program I posted here, so that it can convert negative decimal numbers into binary (that is, two's compliment)... I'll just make it so that when the user enters a minus number, it multiplies that number by minus 1, then convert the positive number it creates into a binary number, then change all the zero's to 1's, and all the one's to 0's, then convert that "flipped" binary number back into decimal, add 1 to that decimal, then convert that decimal back into binary. Easy stuff really. I just need to actually implement it in code
- I currently don't have an idea as to how to do this, but I'll write something that converts a two's compliment binary string to decimal (the user specifies that it's two's complement by prefixing the binary string they enter with a "t"; again, I'll implement this into the program that converts a postive binary number to a decimal
- when I've done both of the above, I'll merge both programs together, and write some code to give the user a little text menu so they can select what they want to do (bin2dec or dec2bin).
- After doing all three of the above, I will implement into this one program, the following things: to ability to do binary addition, multiplication, subtraction, and division.
- After doing all of this, I will try to write this whole program again, but in C++.
As of now, I mother fucking love programming.
Decimal to Binary:
Code: Select all
Module Module1
Sub Main()
Dim lp As Double
Dim digit As Double
Dim mem As Double
Dim binr As String
Dim BinaryString As String
Dim pos As Double
Dim master As Double
master = 1
Do While master = 1
digit = 0
binr = ""
BinaryString = ""
pos = 0
Console.WriteLine("Enter a number to convert to binary: ")
digit = Console.ReadLine()
lp = 1
Do While lp = 1
digit = digit / 2
mem = digit
digit = digit - Int(digit)
If digit > 0 Then
binr = binr + "1"
End If
If digit = 0 Then
binr = binr + "0"
End If
digit = mem
digit = Int(digit)
If digit = 0 Then
lp = 0
End If
Loop
pos = binr.Length
lp = 2
Do While lp = 2
BinaryString = BinaryString + Mid(binr, pos, 1)
pos = pos - 1
If pos = 0 Then
lp = 0
End If
Loop
Console.WriteLine(BinaryString)
Loop
End Sub
End Module
Code: Select all
Module Module1
Sub Main()
Dim pos As Double
Dim BinaryString As String
Dim binr As String
Dim lp As Double
Dim dec As Double
Dim master As Double
Dim negative As Double
master = 1
Do While master = 1
pos = 0
BinaryString = ""
binr = ""
dec = 0
Console.WriteLine("Enter bin: ")
BinaryString = Console.ReadLine()
lp = 1
pos = BinaryString.Length
Do While lp = 1
binr = binr + Mid(BinaryString, pos, 1)
pos = pos - 1
If pos = 0 Then
lp = 0
End If
Loop
pos = 1
lp = 1
Do While lp = 1
If Mid(binr, pos, 1) = "1" Then
dec = dec + (2 ^ (pos - 1))
End If
If Mid(binr, pos, 1) = "0" Then
dec = dec
End If
pos = pos + 1
If pos > binr.Length Then
lp = 0
End If
Loop
Console.WriteLine(dec)
Loop
End Sub
End Module
I'm going to be writing a few more programs in vb soon:
- I'll improve upon the dec2bin program I posted here, so that it can convert negative decimal numbers into binary (that is, two's compliment)... I'll just make it so that when the user enters a minus number, it multiplies that number by minus 1, then convert the positive number it creates into a binary number, then change all the zero's to 1's, and all the one's to 0's, then convert that "flipped" binary number back into decimal, add 1 to that decimal, then convert that decimal back into binary. Easy stuff really. I just need to actually implement it in code
- I currently don't have an idea as to how to do this, but I'll write something that converts a two's compliment binary string to decimal (the user specifies that it's two's complement by prefixing the binary string they enter with a "t"; again, I'll implement this into the program that converts a postive binary number to a decimal
- when I've done both of the above, I'll merge both programs together, and write some code to give the user a little text menu so they can select what they want to do (bin2dec or dec2bin).
- After doing all three of the above, I will implement into this one program, the following things: to ability to do binary addition, multiplication, subtraction, and division.
- After doing all of this, I will try to write this whole program again, but in C++.
As of now, I mother fucking love programming.
these comments are on the top program.
the variable "master" serves no purpose. either give it a purpose or just nuke it. (do... instead of do while...)
instead of the bizarreness of the original being a double and you casting it as an int to get integer division, use the mod function.
actually, you could condense it all down to:
also, if memory serves me correctly, there's a builtin to insert a character at the beginning of a string - that would negate the need for the flipper loop at the end.
edit: and ALWAYS validate your input.
the variable "master" serves no purpose. either give it a purpose or just nuke it. (do... instead of do while...)
instead of the bizarreness of the original being a double and you casting it as an int to get integer division, use the mod function.
actually, you could condense it all down to:
Code: Select all
...
dim digit as integer
...
Do
binr = ""
BinaryString = ""
Console.WriteLine("Enter a number to convert to binary: ")
digit = Console.ReadLine()
Do While digit > 1
binr = binr + (digit % 2)
digit = digit / 2
Loop
binr = binr + digit
pos = binr.Length
Do While pos > 0
BinaryString = BinaryString + Mid(binr, pos, 1)
pos = pos - 1
Loop
Console.WriteLine(BinaryString)
Loop
edit: and ALWAYS validate your input.
Last edited by odditude on Sat Oct 18, 2008 12:57 am, edited 1 time in total.
Why yes, my shift key *IS* broken.
-
- ZSNES Shake Shake Prinny
- Posts: 5632
- Joined: Wed Jul 28, 2004 4:15 pm
- Location: PAL50, dood !
Complicated. You want to make sure every param/input value is the proper type and within boundaries, and if it's not, warn about it and stop execution or replace with proper defaults.
You also might want to warn in case there are too many or too few params. If you don't have defaults, too few must make your program stop gracefully, and so on.
Like...
Let's say prog reads 'filename1' and copies it 'integer' times into a file named 'filename2' (useless as it gets, but it's just an example)
Depending how you convert it from string to integer, you might have to check if integer is positive or not.
Filename1 has to exist to be read. No defaults make it that the program just exits saying "no input file, moron" if you don't give one.
Filename2 might exist, so you'll want added checks to overwrite the file if it does.
Oh, these 2 names must also be stored in buffers long enough to fit them. Each OS has his own limit for that.
If less than 3 params are passed on the CL, you want to either stop or ask for the missing ones (yeah right). If more than 3, depends your take on things.
That's just for those. Each input/param must be sanitized before you can trust it, else you open yourself to overflow exploits and stupid shit like that.
You also might want to warn in case there are too many or too few params. If you don't have defaults, too few must make your program stop gracefully, and so on.
Like...
Code: Select all
$ ./prog <integer> <filename1> <filename2>
Depending how you convert it from string to integer, you might have to check if integer is positive or not.
Filename1 has to exist to be read. No defaults make it that the program just exits saying "no input file, moron" if you don't give one.
Filename2 might exist, so you'll want added checks to overwrite the file if it does.
Oh, these 2 names must also be stored in buffers long enough to fit them. Each OS has his own limit for that.
If less than 3 params are passed on the CL, you want to either stop or ask for the missing ones (yeah right). If more than 3, depends your take on things.
That's just for those. Each input/param must be sanitized before you can trust it, else you open yourself to overflow exploits and stupid shit like that.
皆黙って俺について来い!!
Pantheon: Gideon Zhi | CaitSith2 | Nach | kode54
Code: Select all
<jmr> bsnes has the most accurate wiki page but it takes forever to load (or something)
Well grin, thanks for your advice. I really do need to read up more on these things (also, I don't yet know how to do file input/output).
In any case, I've been messing around with for loops in vb lately.
I decided to rewrite that bin2dec program I posted earlier, in a much cleaner and efficient manner, and this is the result:
Man, the bin2dec program I initially posted was a complete mess.
I'm going to try do the same with my dec2bin program.
EDIT: Here's the new version of my dec2bin program:
(This one was a little harder to cleanup, but it's still cleaner than the last version I posted)
Screw while loops. For loops are much more powerful.
In any case, I've been messing around with for loops in vb lately.
I decided to rewrite that bin2dec program I posted earlier, in a much cleaner and efficient manner, and this is the result:
Code: Select all
Module Module1
Private BinaryString As String
Private digit As Double
Sub Main()
bin2dec()
End Sub
Private Sub bin2dec()
Do
BinaryString = ""
digit = 0
Console.WriteLine("Enter a binary number to convert to decimal: ")
BinaryString = Console.ReadLine()
For pos = BinaryString.Length To 1 Step -1
If Mid(BinaryString, pos, 1) = "1" Then
digit = digit + (2 ^ (BinaryString.Length - pos))
End If
Next
Console.WriteLine(digit)
Loop
End Sub
End Module
I'm going to try do the same with my dec2bin program.
EDIT: Here's the new version of my dec2bin program:
(This one was a little harder to cleanup, but it's still cleaner than the last version I posted)
Code: Select all
Module Module1
Private BinaryString As String
Private digit As Double
Private remain As Double
Private binr As String
Sub Main()
Do
dec2bin()
Loop
End Sub
Private Sub dec2bin()
Console.WriteLine("Enter decimal number to convert to binary: ")
digit = Console.ReadLine()
BinaryString = ""
binr = ""
Do Until digit = 0
remain = (digit / 2) - Int(digit / 2)
If remain > 0 Then
binr = binr + "1"
End If
If remain = 0 Then
binr = binr + "0"
End If
digit = (digit / 2) - remain
Loop
For pos = binr.Length To 1 Step -1
BinaryString = BinaryString + Mid(binr, pos, 1)
Next
Console.WriteLine(BinaryString)
End Sub
End Module
Last edited by ZH/Franky on Sat Oct 18, 2008 6:11 pm, edited 9 times in total.
VB. ugh.
while loops are good for when you have no idea the number of loops before entering the loop.
while loops are good for when you have no idea the number of loops before entering the loop.
Last edited by funkyass on Sat Oct 18, 2008 6:22 pm, edited 1 time in total.
Does [Kevin] Smith masturbate with steel wool too?
- Yes, but don’t change the subject.
- Yes, but don’t change the subject.
-
- Seen it all
- Posts: 2302
- Joined: Mon Jan 03, 2005 5:04 pm
- Location: Germany
- Contact:
It's a bad copy of Delphi. 
EDIT: PM sent.

EDIT: PM sent.
Last edited by creaothceann on Sat Oct 18, 2008 9:49 pm, edited 1 time in total.
vSNES | Delphi 10 BPLs
bsnes launcher with recent files list
bsnes launcher with recent files list
Delphi... you mean pascal?
Anyway, I merged both programs (bin2dec and dec2bin):
All very efficient and easy to understand.
I've seperated the actual algorithms from the input/output commands, which makes it easy for me to implement new things now (such as the ability to convert negative integers to two's compliment binary, do binary addition, binary subtraction, binary multiplication, binary division, etc. Binary square roots! Oh yeah, you know that's cool.)
EDIT:
Not yet perfect, but I made an attempt at implementing negative decimal to two's compliment binary conversion:
I'm not sure whether it's correct though. Am I right in saying that to find a negative decimal in binary you (for example, if you had 12, which in binary is 1100, and you wanted to find the binary value of -12) you would flip the zero's, in effect making 0011, or just 11, and then add 1, in effect creating 0100; that is, -12 = 0100?
As a side note, I rewrote that factorial finder I posted:
E.g.
while loop:
"loop until" loop:
for loop:
Maybe while loop's are cool for certain things, not sure; but I do know that recently, I've found myself writing much cleaner code by not using while loops.
Anyway, I merged both programs (bin2dec and dec2bin):
Code: Select all
Module Module1
Private BinaryString As String
Private digit As Double
Private remain As Double
Private binr As String
Private choice As String
Sub Main()
Do
Console.WriteLine("bin2dec or dec2bin?")
choice = Console.ReadLine()
If choice = "bin2dec" Then
BinaryString = ""
digit = 0
Console.WriteLine("Enter a binary number to convert to decimal: ")
BinaryString = Console.ReadLine()
bin2dec()
Console.WriteLine(digit)
End If
If choice = "dec2bin" Then
Console.WriteLine("Enter decimal number to convert to binary: ")
digit = Console.ReadLine()
BinaryString = ""
binr = ""
dec2bin()
Console.WriteLine(BinaryString)
End If
Loop
End Sub
Private Sub dec2bin()
Do Until digit = 0
remain = (digit / 2) - Int(digit / 2)
If remain > 0 Then
binr = binr + "1"
End If
If remain = 0 Then
binr = binr + "0"
End If
digit = (digit / 2) - remain
Loop
For pos = binr.Length To 1 Step -1
BinaryString = BinaryString + Mid(binr, pos, 1)
Next
End Sub
Private Sub bin2dec()
For pos = BinaryString.Length To 1 Step -1
If Mid(BinaryString, pos, 1) = "1" Then
digit = digit + (2 ^ (BinaryString.Length - pos))
End If
Next
End Sub
End Module
I've seperated the actual algorithms from the input/output commands, which makes it easy for me to implement new things now (such as the ability to convert negative integers to two's compliment binary, do binary addition, binary subtraction, binary multiplication, binary division, etc. Binary square roots! Oh yeah, you know that's cool.)
EDIT:
Not yet perfect, but I made an attempt at implementing negative decimal to two's compliment binary conversion:
Code: Select all
Module Module1
Private BinaryString As String
Private digit As Double
Private remain As Double
Private binr As String
Private choice As String
Private quit As Double
Private negative As Double
Sub Main()
quit = 0
Do Until quit = 1
Console.WriteLine("bin2dec or dec2bin? to quit, enter q")
choice = Console.ReadLine()
If choice = "q" Then
quit = 1
End If
If choice = "bin2dec" Then
Console.WriteLine("Enter a binary number to convert to decimal: ")
BinaryString = Console.ReadLine()
digit = 0
bin2dec()
Console.WriteLine(digit)
End If
If choice = "dec2bin" Then
Console.WriteLine("Enter decimal number to convert to binary: ")
digit = Console.ReadLine()
If digit < 0 Then
digit = digit * -1
negative = 1
End If
BinaryString = ""
binr = ""
dec2bin()
If negative = 1 Then
binr = ""
flipbits()
binr = ""
bin2dec()
digit = digit + 1
dec2bin()
binr = ""
revbin()
negative = 0
End If
Console.WriteLine(BinaryString)
End If
Loop
End Sub
Private Sub dec2bin()
Do Until digit = 0
remain = (digit / 2) - Int(digit / 2)
If remain > 0 Then
binr = binr + "1"
End If
If remain = 0 Then
binr = binr + "0"
End If
digit = (digit / 2) - remain
Loop
For pos = binr.Length To 1 Step -1
BinaryString = BinaryString + Mid(binr, pos, 1)
Next
End Sub
Private Sub bin2dec()
For pos = BinaryString.Length To 1 Step -1
If Mid(BinaryString, pos, 1) = "1" Then
digit = digit + (2 ^ (BinaryString.Length - pos))
End If
Next
End Sub
Private Sub flipbits()
binr = ""
For pos = 1 To BinaryString.Length Step 1
If Mid(BinaryString, pos, 1) = "1" Then
binr = binr + "0"
End If
If Mid(BinaryString, pos, 1) = "0" Then
binr = binr + "1"
End If
Next
BinaryString = binr
End Sub
Private Sub revbin()
For pos = BinaryString.Length To 1 Step -1
binr = binr + Mid(BinaryString, pos, 1)
Next
BinaryString = binr
End Sub
End Module
As a side note, I rewrote that factorial finder I posted:
Code: Select all
Module Module1
Private digit As Double
Sub Main()
Do
Console.WriteLine("Enter number to find factorial of:")
digit = Console.ReadLine()
factorials()
Console.WriteLine(digit)
Loop
End Sub
Private Sub factorials()
If digit > 0 Then
For pos = digit To 2 Step -1
digit = digit * (pos - 1)
Next
End If
If digit < 0 Then
For pos = digit To -2 Step 1
digit = digit * (pos + 1)
Next
If digit > 0 Then
digit = digit * (-1)
End If
End If
End Sub
End Module
Even then, I've found that "loop until" is often much better than "while condition... loop".while loops are good for when you have no idea the number of loops before entering the loop.
E.g.
while loop:
Code: Select all
while whatever = 1 do
blah blah blah
something = something + 1
if something = somethingelse then
whatever = 0
end if
loop
Code: Select all
loop until something = somethingelse
blah blah blah
something = something + 1
next
Code: Select all
for pos = something to somethingelse step 1
blah blah blah
loop
Look into the kinds of integers found in computer science. Here's a hint: are two, signed and unsigned. Once you get outside of whole numbers you are pretty much stuck using a separate set of functions for integers, and another set for real numbers for base conversions.
I even won't think of imaginary numbers.
As for loops, the major difference between structures is when you are checking the loop condition - before or after the block of code. Out of all programing languages, you are guaranteed while as part of its syntax(either by itself, or as a part of do-while variant).
I even won't think of imaginary numbers.
As for loops, the major difference between structures is when you are checking the loop condition - before or after the block of code. Out of all programing languages, you are guaranteed while as part of its syntax(either by itself, or as a part of do-while variant).
Does [Kevin] Smith masturbate with steel wool too?
- Yes, but don’t change the subject.
- Yes, but don’t change the subject.
Well, I think I remember reading that an unsigned integer is one that can be between 0 and 255 (that is, it's within an 8-bit address space), and that a signed integer is one that that can be between -255 and +255. That is, for example, 28 in an 8-bit address space would be 00011100, and -28 would be 11100100.Look into the kinds of integers found in computer science. Here's a hint: are two, signed and unsigned. Once you get outside of whole numbers you are pretty much stuck using a separate set of functions for integers, and another set for real numbers for base conversions.
Am I correct?
So is "unsigned and signed" limited to 8-bit? Or does it have the be specified exactly how many bits you want to work with?
It all looks quite easy to understand; I just need to clear up some of the confusion.
-
- Seen it all
- Posts: 2302
- Joined: Mon Jan 03, 2005 5:04 pm
- Location: Germany
- Contact:
Integers can be signed or unsigned. "signed" means that half the range is in the negative domain.Wikipedia wrote:The integers are the set of numbers consisting of the natural numbers including 0 (0, 1, 2, 3, ...) and their negatives (0, −1, −2, −3, ...). They are numbers that can be written without a fractional or decimal component, and fall within the set {... −2, −1, 0, 1, 2, ...}. For example, 65, 7, and −756 are integers; 1.6 and 1½ are not integers.
Code: Select all
byte = 8-bit integer: range of 2^8 = 256 values
word = 16-bit integer: range of 2^16 = 65536 values
double word = 32-bit integer: range of 2^32 = 4294967296 values
unsigned byte = 0.. 255
unsigned word = 0.. 65535
unsigned dword = 0..4294967295
signed byte = -128..127
signed word = -32768..32767
signed dword = -2147483648..2147483647
vSNES | Delphi 10 BPLs
bsnes launcher with recent files list
bsnes launcher with recent files list
-
- Buzzkill Gil
- Posts: 4295
- Joined: Wed Jan 12, 2005 7:14 pm
*shakes head*Franky wrote:Well, I think I remember reading that an unsigned integer is one that can be between 0 and 255 (that is, it's within an 8-bit address space), and that a signed integer is one that that can be between -255 and +255. That is, for example, 28 in an 8-bit address space would be 00011100, and -28 would be 11100100.Look into the kinds of integers found in computer science. Here's a hint: are two, signed and unsigned. Once you get outside of whole numbers you are pretty much stuck using a separate set of functions for integers, and another set for real numbers for base conversions.
Am I correct?
Think of the range. An 8-bit sequence can represent 256 unique states.
-255 to +255 represents almost twice as many states. You need a 9th bit for -255 to +255.
9-bit values are highly uncommon, for various reasons.
An 8-bit signed integer represents -128 to +127.
http://en.wikipedia.org/wiki/Integral_d ... type_namesSo is "unsigned and signed" limited to 8-bit? Or does it have the be specified exactly how many bits you want to work with?
While not limited to 1-byte values, the specifics depend on the language.
-
- Seen it all
- Posts: 2302
- Joined: Mon Jan 03, 2005 5:04 pm
- Location: Germany
- Contact:
And the architecture (16-, 32- or 64-bit CPU).
vSNES | Delphi 10 BPLs
bsnes launcher with recent files list
bsnes launcher with recent files list